OpenSCAD is an excellent tool for programmatically generating complex geometries. However, creating rounded or organic shapes often requires writing extensive amounts of code. PolyEdge is a compact module for OpenSCAD designed to simplify this process by enabling users to generate polygons with customizable corners. Each corner can be specified as either rounded, standard, or inset.
Syntax
PolyEdge generates polygons in a manner similar to the polygon() function in OpenSCAD, with the addition of an optional third parameter, t, for each point. This parameter controls the behavior of each corresponding edge, offering the flexibility to define edges as rounded, standard, or inset.
polyedge([ [x, y, t], ...]);
The parameter t has the following options:
- = 0: If t is zero (or left out), the edge is becoming a normal sharp edge like in polygon()
- > 0: If t is positive, the edge will get a round corner with radius t
- < 0: If t is negative, the edge will get an inset of length -t from the original edge
Simple Examples
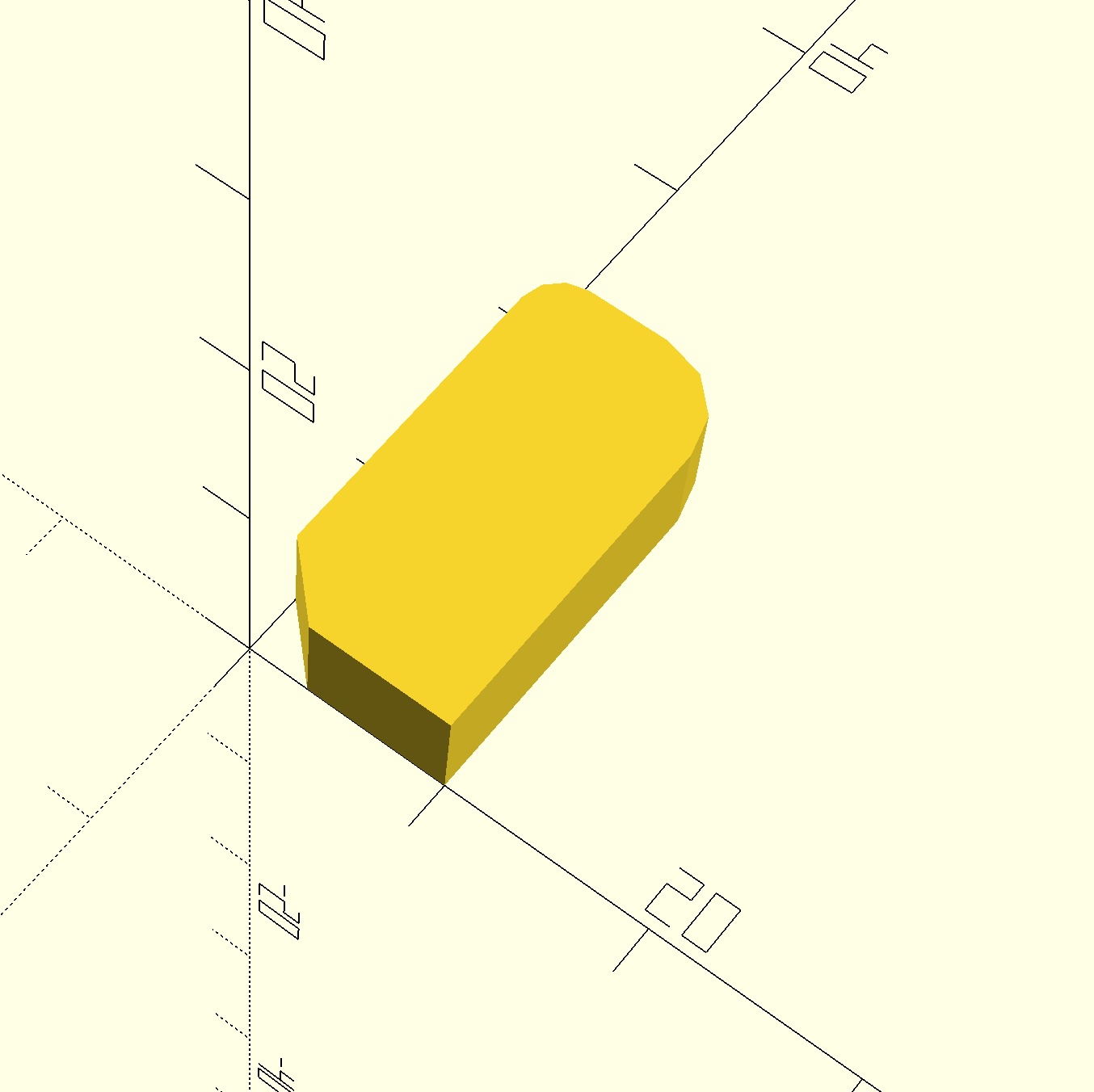
include <polyedge.scad>
linear_extrude(height = 5)
polyedge([
[0, 0, -3], // First corner with inset of 3
[10, 0, 0], // Go to the right and draw a standard edge
[10, 20, 4], // Go up and draw a rounded corner with radius 4
[0, 20, 2], // Go to the left and draw a rounded corner with radius 2
]);
Wood Stick Center Finder
A more complex example is a tool to find the center of a stick. It's pretty straightforward to define the object using only 3 polyedges.
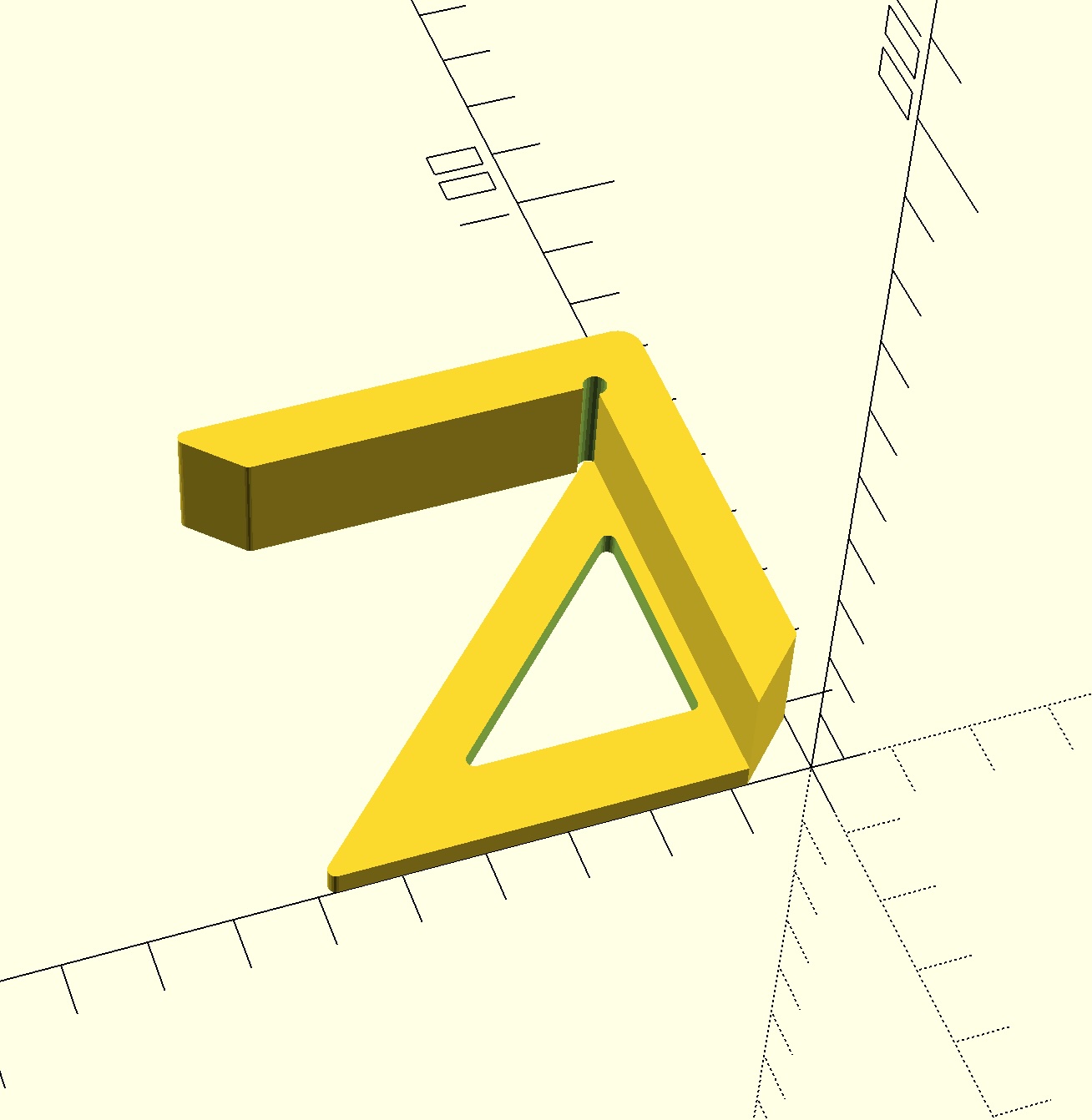
include <polyedge.scad>
$fn = 20;
width = 60;
height = 15;
edgeRadius = 1;
wallWidth = 8;
notchWallWidth = 10;
notchRadius = 1.5;
difference() {
union() {
linear_extrude(height=height)
polyedge([
[0, wallWidth, 0],
[wallWidth, 0, edgeRadius],
[width, 0, 3],
[width, width, edgeRadius],
[width - wallWidth, width - wallWidth, edgeRadius],
[width - wallWidth, wallWidth, 0],
]);
linear_extrude(height=3)
polyedge([
[0, wallWidth, 0],
[wallWidth, 0, edgeRadius],
[width, 0, notchRadius],
[0, width, edgeRadius]
]);
}
translate([width - wallWidth, wallWidth, -1])
cylinder(r=notchRadius, h=height + 2);
translate([0,0,-1])
linear_extrude(height=height)
polyedge ([
[notchWallWidth, notchWallWidth, edgeRadius],
[notchWallWidth, width - notchWallWidth * 2, edgeRadius],
[width - notchWallWidth * 2, notchWallWidth, edgeRadius]
]);
}